How to work remotely and Earn Dollars with Programming – pt 4
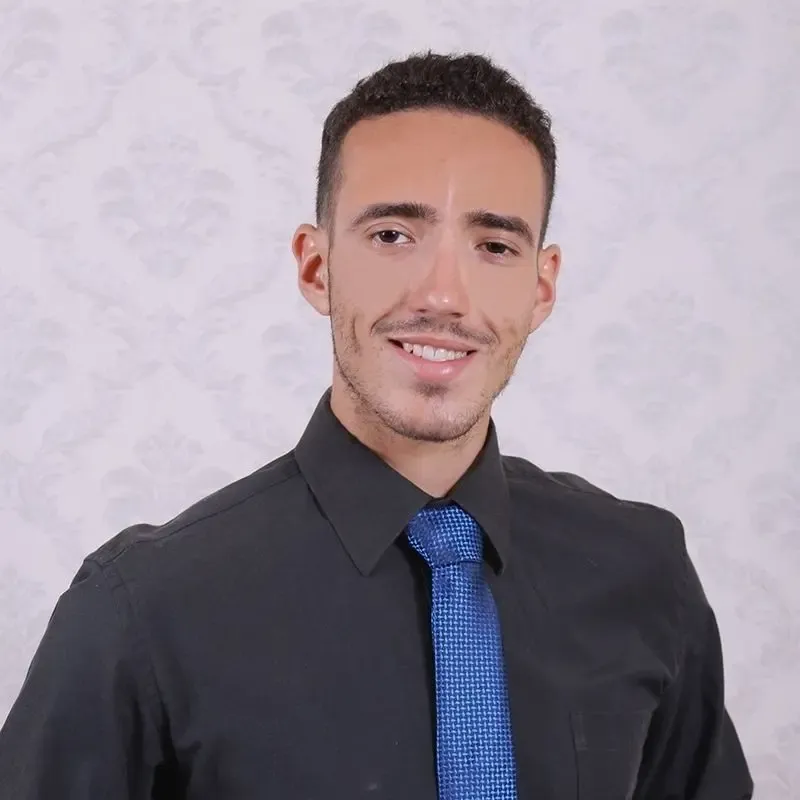
When applying for a software development position, possibly you will be required to perform a code challenge, and investing some preparation time here is important.
The code challenge preparation goes beyond just programming, it involves several aspects I will address below, including:
- Understand the question command;
- Time management;
- Structure a solution;
- Test the developed algorithm;
- Explain the approach used.
First, find here a image showing the general structure of the code challenge environment:
Fig 1. Example of a code challenge environment
In [Fig. 1] we can see that the code challenge environment is composed of the following elements:
- Command of the question: the most important aspect since without a full understanding of the problem, it will be impossible to solve the algorithm properly.
- Choice of programming language: in general, code challenge platforms offer a wide variety of programming languages. The main ones are Java, Javascript, PHP, C, C++, and others. Important: if you choose to change the programming language in the middle of the test, all the previous work will be lost, so choose the language carefully before starting to program and avoid changing it!
- Code editor: the part in which you will spend most of the time. In general, the editors are modern, with row and column counters, some even include the programming language’s auto-completion function, but do not rely on this option.
- Buttons for submitting the developed code: at the end of the algorithm or even during its development, you can always check if your code is compiling correctly and if is passing in the tests.
After this overview about the code challenge environment, let’s go to the crucial points regarding the preparation for the test.
Understand the question command!
Before skipping steps and going into the coding mode, take a few minutes to understand what the question is all about.
Fig 2. Question command sections
Observing the [Fig. 2], you will notice that the question statement is divided into sections beyond the introductory paragraph, including:
- Input/output format: Once you understand the general context of the problem, you will need to know how the data will be input and output. In this section, you will receive precisely what these formats are. In the case of [Fig. 2], the problem states that the input will consist of 4 lines, the first will be an integer representing age, the second will be a lowercase string representing the student’s name, the third will also be a string lowercase representing the student’s last name, and finally, the last line will be an integer representing the average of their grades. Additionally, a note indicates that the strings will not exceed more than 50 characters.
- Sample input/output: Here, the question statement will give you an example of both the input and output of data, namely:
Input
15 = age
john = name
carmack = surname
10 = average of grades
Output
15
carmack, john
10
15, john, carmack, 10
Understanding how to receive, process, and return data is crucial here. Without this understanding, you will not be able to progress through the problem. I usually invest the first 5 minutes of the code challenge to understand the problem.
Time management
- First 5 minutes: focus on understanding the problem;
- Next 5 minutes: mentally organize the solution with a paper and a pen;
- Next 35 minutes: code the first version of the algorithm, as simple as possible, and improve if there is time. An important tip:
- Always run the program to see if it is compiling correctly and get ‘typos’ right away;
- Last 15 minutes: Verify if the algorithm passes in all available test cases. Usually, the algorithm passes only in some tests, but not others. Try to use this final time to make the last adjustments.
Note: I’m assuming that the code challenge model you came across doesn’t have an item asking you to explain your approach, as well as the asymptotic complexity of your algorithm. Be aware that this may show up on your test!
Structure a solution
Once you understand the problem statement, now is the time to get your hands dirty!
A very efficient method for solving problems is the Pólya method. It is based on a few steps:
- Understand the problem (done!)
- Make a plan
- Execute the plan
- Look back
In practice, the plan elaboration can be a draft of the solution (pseudocode).
Pseudocode is a simple description of the steps in an algorithm. In other words, your pseudocode is your step-by-step plan for how to solve the problem.
Write down the steps you need to take to solve the problem. For a more complicated problem, you will include more steps. Here’s an example:
// Create a sum variable.
Add the first input to the second input using the addition operator.
// Store value of both inputs into sum variable.
// Return as output the sum variable.
Now that you have a step-by-step plan for solving the problem, it’s time to code. Unfortunately in this part, I can’t help you much, you will need to be sharp on your chosen programming language to avoid wasting time trying to remember the syntax of commands. That’s why I recommend a lot of training before starting the code challenge!
Use Hackerrank and Leetcode platforms to practice in advance.
Test the developed algorithm
Now that we’ve reached the final part of the code challenge, it’s time to submit our work to the test! Therefore, the last step is to test the developed algorithm and see if it passed the tests. [Fig. 3] shows examples of test results:
Fig 3. Examples of algorithms that failed and passed the tests:
If one or more tests fail equally to [Fig. 3A] after running them, you will need to check the specific test that failed by clicking the tab corresponding to it. Unfortunately, some of the tests are hidden and have a lock, so you can not see the complete input data. Therefore, it is essential to plan your algorithm before coding it, thinking about possible errors.
If all tests are successfully equal to [Fig. 3B], congratulations! You are ready to submit your solution. If there isn’t an extra question asking you to explain the approach used in your algorithm, use the remaining time you have to polish your code.
Explain the approach used
On rare occasions, I’ve come across extra questions asking to explain the approach used in the algorithm, as well as its asymptotic complexity. If you don’t understand this concept well, I strongly suggest you study it before starting the code challenge. You can start with this link on asymptotic complexity.
To go further
If you want to learn in-depth the algorithms concepts, data structure, and algorithm complexity, you can find below some book recommendations:
Estruturas de Dados e Seus Algoritmos | Cracking the Coding Interview: 189 Programming Questions and Solutions | Introduction to Algorithms |
![]() | ![]() | ![]() |
In the next post, I will talk about the mini-project stage (take home) that may appear in some selective processes.
See you next time!
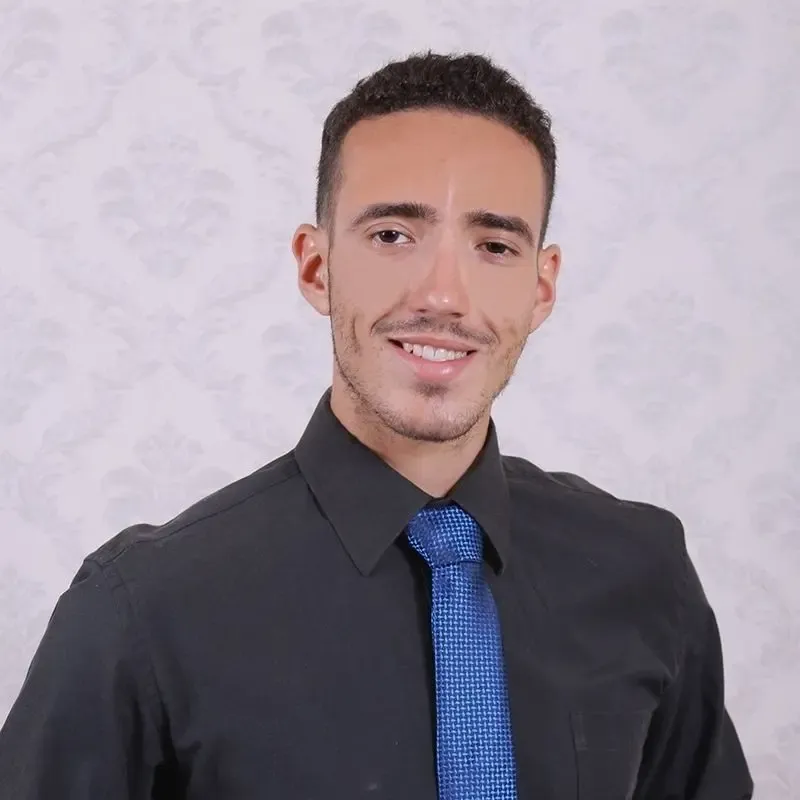
Igor Augusto Brandão
#igorabrandaoHello, world! My name is Igor and I am a system analyst professional with degrees in Systems Analysis, Business Administration, Information Technology, a specialization of IT applied to the Legal Area, an MSc in Bioinformatics, and +10 years of experience working with systems development.